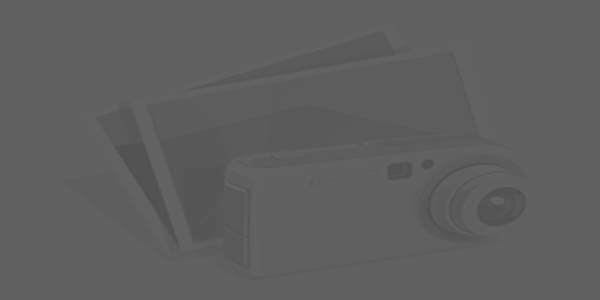
C Language Programs
C Programming Examples
This page contains the list of C programming examples which covers the concepts like basic c programs, programs on numbers, loop programs, functions, recursions etc.
All the C programming examples that are present in this page might contain at least three examples, which includes program using For Loop, using While Loop, Functions. In some case, Recursions.
Simple C Programming Examples
The following C programming examples are the basic and simple programs.
Please visit our C Language section to learn C Programming with examples
- C Program to Print Hello World
- C Program to Add Two Numbers
- C Program for Average of Two Numbers
- C program to find Compound Interest
- C program to Calculate Cube of a Number
- C Program to check Number is Even or Odd
- C Program to Print Even Numbers from 1 to N
- C Program to find Largest of Two Numbers
- C Program to find Largest of Three Numbers
- C Program to print Multiplication Table
- C program to find Number is Divisible by 5 and 11
- C Program for nCr Calculation
- C Program for nPr Calculation
- C Program to print Odd Numbers from 1 to N
- C program to calculate Power of a Number
- C program to find Profit or Loss
- Print an Integer, Character, and Float Value
- C Program to find the size of int, float, double, and char
- Positive or Negative Number
- Print 1 to 100 without using Loop
- Roots of a Quadratic Equation
- Simple Interest
- Standard Deviation
- Sum of Even and Odd numbers in a Given Range
- Square of a Number
- Square root of a Number
- C Program to Print First 10 Even Natural Numbers
- C Program to Print First 10 Natural Numbers
- C Program to Print First 10 Natural Numbers in Reverse
- C Program to Print First 10 Odd Natural Numbers
- Sum of Even Numbers from 1 to n
- Sum of Odd Numbers from 1 to n
- Student Grade
- Total, Average, and Percentage of Five Subjects
- C example to accept User Input and Print
C Programming Examples on Numbers
The list of advanced Number programs in C Programming language with multiple examples.
- C Program to check the Number is Armstrong Number
- C program to Count Number of Digits in a Number
- C program to Check Number is a Prime, Armstrong, or Perfect Number
- C program to Calculate Electricity BillC
- C Program to find Factors of a Number
- C Program to find Factorial of a Given Number
- C program to print First Digit of Number
- C program to print First and Last Digit of a Number
- C program to calculate GCD of Two Numbers
- C program to calculate Generic Root of a Number
- C program to find Gross Salary of an Employee
- C program to print Last Digit of Number
- C program to calculate LCM of Two Numbers
- C program to find NCR Factorial of a Number
- C program to print Natural Numbers from 1 to N
- C program to print Natural Numbers in Reverse Order
- C program to Check Palindrome or Not
- C program to check Perfect Number or Not
- C program to check Prime Number
- C program to print Prime Numbers from 1 to 100
- C program, to calculate Product of Digits of a Number
- C program to find Prime Factors of a Number
- C example to Reverse a Number
- C example to Swap Two Numbers
- C example to fid Sum of First and Last Digit of a Number
- C example to Swap First and Last Digit of a Number
- C example to find Sum of N Natural Numbers
- C example to calculate Sum and Average of N Numbers
- C program to check Strong Number
- C program to find Sum of Digits in a Given Number
- C program for Simple Calculator
- Total Notes in a Given Amount
Calendar Programs in C
- C Program to find the Leap Year
- C example to find the Day Name of a Week
- Days to Years Weeks and Days
- Number of Days in a Month
Conversion Programs in C
The following are the list of C programs to Convert
- C Program to Convert Binary to Decimal
- C example to Convert Centimeter to Meter and Kilometer
- Celsius to Fahrenheit
- Decimal to Binary Number
- Decimal to Octal Number
- Fahrenheit to Celsius
- Kilometer to Meter Centimeter and Millimeter
C Programs on Characters
Below programs are the C Programs to Check whether the Character is
- C Program to find the ASCII Value of Single Character
- C example to find ASCII Values of all Characters
- Alphabet or Not
- Alphabet or Digit
- Convert Character to Uppercase
- Convert Character to Lowercase
- Digit or Not
- Digit or Alphabet or Special Character
- Lowercase or Not
- Lowercase or Uppercase Alphabet
- Print Alphabets from a to z
- Print Alphabets between A and Z
- Uppercase or Not
- Vowel or Consonant
C Programming Examples on String
- C Program to find the ASCII Value of Total Characters in a String
- C example to find All Occurrence of a Character in a String
- C programming example to find Characters in a String
- Compare two Strings
- Concatenate Two Strings
- Copy String
- Count Alphabets, Digits and Special Characters in a String
- Count Vowels, and Consonants in a String
- Counting All Occurrence of a Character in a String
- Count Total Number of Words in a String
- Frequency of each Character in a String
- First Occurrence of a Character in a String
- First Occurrence of a Word in a String
- Last Occurrence of a Character in a String
- Length of a String
- Lowercase to Uppercase
- Maximum Occurring Character in a string
- Minimum Occurring Character in a String
- Palindrome String
- Remove First Occurrence of a Character in a String
- Remove Last Occurrence of a Character in a String
- Removing All Occurrences of a Character in a String
- Remove All Duplicate Characters in a String
- Replace First Occurrence of a Character in a String
- Replace Last Occurrence of a Character in a String
- Replacing All Occurrence of a Character in a String
- Reverse a String
- Reverse Order of Words in a String
- Toggle Case of all Characters in a String
- Uppercase to Lowercase
C Programming Examples on Area
The following are the list of Area programs in C language
- C Program to Find the Area of a Circle
- Diameter, Circumference, and Area of a Circle
- Area of a Triangle
- Area of a Triangle using Base and Height
- Angle of a Triangle if two angles are given
- Triangle is Equilateral Isosceles or Scalene
- Use Angles to check Triangle is valid or Not
- Use Sides to check Triangle is Valid or Not
- Area of an Isosceles Triangle
- Area of Rectangle
- Area of a Rectangle using Length and Width
- Perimeter of a Rectangle using Length and Width
- Area of a Parallelogram
- Area of a Trapezoid
- Area of a Right Angled Triangle
- Find Area of an Equilateral Triangle
- Area of a Rhombus
- Perimeter of a Rhombus
Volume and Surface Area Programs in C
Following are the list of Volume and Surface Area programs
- Volume and Surface Area of Sphere
- Volume and Surface Area of a Cylinder
- Find Volume and Surface Area of a Cube
- Volume and Surface Area of a Cuboid
- Volume and Surface Area of a Cone
Array Programs in C
The following are the c programming examples on Arrays
- C Program to Perform Arithmetic Operations on One Dimensional Array
- C Program to find the Number of Elements in an Array
- C example to Count Even and Odd Numbers in an Array
- C example Count Positive and Negative Numbers in an Array
- C example to Copy an Array to another
- Count Frequency of each Element in an Array
- Count Total Number of Duplicate Elements in an Array
- Delete an Element in an Array
- Delete Duplicate Elements from an Array
- Insert an Element into an Array
- Largest Number in an Array
- Largest and Smallest Number in an Array
- Length or Size of an Array
- Merge Two Arrays
- Print Elements in an Array
- Put Even and Odd Numbers in two Separate Arrays
- Put Positive and Negative Numbers in two Separate Arrays
- Print Negative Numbers in an Array
- Print Positive Numbers in an Array
- Reverse an Array
- Search for Element in an Array
- Second largest Number in an Array
- Smallest Number in an Array
- Sort Array in Ascending Order
- Sort Array in Descending Order
- Sum of all Elements in an Array
- Sum of Even and Odd Numbers in an Array
- Swap Two Arrays Without Using Temp Variable
- Unique Elements in an Array
Matrix Programs
- C Program to Perform Arithmetic Operations on Multi-Dimensional Arrays
- C example to add two Matrices
- C example to Check Two Matrices are Equal or Not
- C example to Determinant of a Matrix
- Identity Matrix
- Interchange Diagonals of a Matrix
- Lower Triangle Matrix
- Scalar Multiplication of a Matrix
- Sparse Matrix
- Sum of Diagonal Elements in a Matrix
- Sum of each and every Row and Column in a Matrix
- Summing each row in a Matrix
- Sum of each column in a Matrix
- Subtract Two Matrices
- Sum of Lower Triangle Matrix
- Sum of Opposite Diagonal Elements in a Matrix
- Symmetric Matrix
- Sum of Upper Triangle Matrix
- Transpose a Matrix
- Upper Triangle Matrix
C Sort Examples
Pointers Programs
C Programs to print Series
- Fibonacci Series Program
- Find Nth Fibonacci Number
- Sum of series 1²+2²+3²+….+n²
- Sum of series 1³+2³+3³+….+n³
- Find Sum of Geometric Progression Series
- Sum of Arithmetic Progression Series
C Programs to display Patterns and Shapes
The following are the list of C programs to Print patterns and shapes
- C Program to Print Christmas Tree Star Pattern
- C Example to print exponentially Increasing Star Pattern
- C example to Print Floyd’s Triangle
- C example to Print Diamond Star Pattern
- C example to Print Half Diamond Star Pattern
- C example to Print Mirrored Half Diamond Star Pattern
- C example to Print Left Arrow Star Pattern
- C example to Print Pascal Triangle
- C example to Print Pyramid Star Pattern
- C example to Print Hollow Pyramid Star
- C example to Print Inverted Pyramid Star Pattern
- C example to Print Hollow Inverted Star Pyramid
- Plus Star Pattern
- Rectangle Star Pattern
- Hollow Rectangle Star Pattern
- Right Arrow Star Pattern
- Right Angled Triangle Star Pattern
- Hollow Right Triangle Star Pattern
- Reversed Mirrored Right Triangle
- Mirrored Right Triangle Star Pattern
- Hollow Mirrored Right Triangle Star Pattern
- Inverted Right Triangle Star Pattern
- Hollow Inverted Right Triangle Star Pattern
- Inverted Mirrored Right Triangle Star Pattern
- Hollow Inverted Mirrored Right Triangle Star Pattern
- Rhombus Star Pattern
- Hollow Rhombus Star Pattern
- Mirrored Rhombus Star Pattern
- Hollow Mirrored Rhombus Star Pattern
- Square Star Pattern
- Hollow Square Star Pattern
- Hollow Square Pattern With Diagonals
- C Program to Print Hollow Sandglass Star Pattern
- C Program to Print H Star Pattern
- C Program to Print X Star Pattern
- C Program to Print Inverted V Star Pattern
- C Program to Print W Star Pattern
- C Program to Print 8 Star Pattern
C Alphabet Pattern Programs
- C Program to Print Diamond Alphabets Pattern
- C Program to Print Downward Triangle Alphabets Pattern
- C Program to Print Downward Triangle Mirrored Alphabets Pattern
- C Program to Print Inverted Right Triangle Alphabets Pattern
- C Program to Print Inverted Triangle Alphabets Pattern
- C Program to Print K Shape Alphabets Pattern
- C Program to Print Left Arrow Alphabets Pattern
- C Program to Print Mirrored Right Triangle Alphabets Pattern
- C Program to Print Pyramid Alphabets Pattern
- C Program to Print Right Triangle Alphabets Pattern
- C Program to Print Same Alphabet in each Right Triangle Column
- C Program to Print Triangle Alphabets Pattern
C Programming Examples on Number pattern
The following are the remaining number pattern C programming examples.
- C program to Print Box Number Pattern of 1 and 0
- C Program to Print Downward Triangle Mirrored Numbers Pattern
- C example to Print Hollow Box Number
- C example to Print Inverted Right Triangle Number Pattern
- C Program to Print Inverted Triangle Numbers Pattern
- C example to Print 1 and 0 in Alternative Rows
- C example to Print 1 and 0 in Alternative Columns
- C Program to Print Left Arrow Numbers Pattern
- C Program to Print Pyramid Numbers Pattern
- C example to Print Right Triangle Number Pattern
- C Program to Print Consecutive Column Numbers in Right Triangle
- C Program to Print Consecutive Row Numbers in Right Triangle
- C Program to Print Numeric Right Triangle Pattern 2
- C Program to Print Numeric Right Triangle Pattern 3
- C Program to Print Right Triangle of Incremented Numbers
- C program to print Right Triangle of Numbers in Decreasing order
- C Program to print Square Number Pattern
- C Program to Print Square where each row contains one Number
- C Program to Print Square where each column contains one Number
- C Program to Print Same Numbers in Rows and Columns
- C Program to Print K Shape Number Pattern
- C program to Print Sandglass Number Pattern
0 Response to "C Language Programs"
Post a Comment